421 days later :-) ( some coding elements, in JavaScript for Applications, for reference )
Example: setting the background color of each circle on the canvas according to the number in the circle’s text.
(here, choosing one of 8 colors, ordered by saturation (taken from ColorBrewer), to match the position of the number on a scale 1-100)
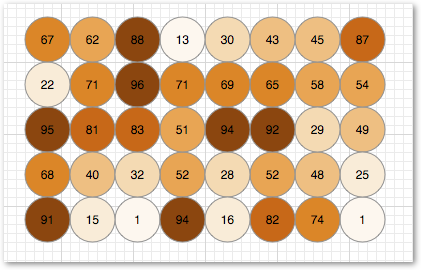
(function () {
'use strict';
// TESTING: write a random number (in given range)
// into the text of a shape
function numberShape(shp, min, max) {
min = min || 0,
max = max || 100;
var n = Math.floor(Math.random() * (max - min + 1)) + min;
shp.text = n.toString();
}
// Choose, from a set of ordered colors of arbitrary length
// the color corresponding to the position of a number within a range
function numberColor(n, min, max, lstColors) {
var lngColors = lstColors.length,
i = Math.ceil(((n - min) * lngColors) / (max - min)) - 1;
return lstColors[
(i >= 0) && (i <= (lngColors - 1)) ? i : 0
];
}
// rewrite a triple of integers (0-255)
// as a triple of reals between 0 and 1
function rgb(r, g, b) {
return [r / 255, g / 255, b / 255];
}
var og = Application('OmniGraffle'),
ws = og.windows.whose({
_not: [{
document: null
}]
}),
w = ws.length ? ws.at(0) : undefined,
cnv = w ? w.canvases.at(0) : undefined;
// A range of 8 colors taken from http://colorbrewer2.org/
var lstPalette = [[1, 0.9607843137254902, 0.9215686274509803], [
0.996078431372549, 0.9019607843137255, 0.807843137254902], [
0.9921568627450981, 0.8156862745098039, 0.6352941176470588], [
0.9921568627450981, 0.6823529411764706, 0.4196078431372549], [
0.9921568627450981, 0.5529411764705883, 0.23529411764705882], [
0.9450980392156862, 0.4117647058823529, 0.07450980392156863], [
0.8509803921568627, 0.2823529411764706, 0.00392156862745098], [
0.5490196078431373, 0.17647058823529413, 0.01568627450980392]];
// TO TEST CODE BELOW:
// PLACE RANDOM NUMBERS 1-100 IN EACH CIRCLE ON THE CANVAS
var lstShapes = cnv.shapes.whose({
_match: [ObjectSpecifier().name, "Circle"]
}).callAsFunction().map(function (x) {
numberShape(x);
return x;
});
// MAIN: setting the fill color according to the number in the text
// RANGE OF EXPECTED NUMBERS ?
var min = 1,
max = 100;
// TYPE OF SHAPE CONTAINING NUMBERS ?
var strShapeType = "Circle";
// PROPERTY OF SHAPE TO BE COLORED ?
var strColorProperty = 'fillColor'; // or 'strokeColor' etc
// DEFINE A SET OF SHAPES, AND ITERATE THROUGH IT
// CONDITIONALLY SETTING A COLOR PROPERTY
cnv.shapes.whose({
_match: [ObjectSpecifier().name, strShapeType]
})() // call the reference as a function to get an array
// and iterate through the array setting fill colors by number
.forEach(function (shp) {
shp[strColorProperty] = numberColor(
parseFloat(shp['text']()),
min, max,
lstPalette
);
});
})();